while
is another looping statement in java which allows programmers to repeat the execution of
one or more line of code as far as a condition is true
. The computer keep repeating the execution of statements
inside the while
loop until the condition get's false
. Programmer can also read this as " execute given
statements while
something is true
". The syntax of while
loop is :
while
(condition) {// code to be executed inside while loop
}
Here condition is a boolean expression which must return either true
or
false
. If it returns true
, the code written inside
while
block get's
executed and if it returns false
, while
block code will not be executed. Everything that comes inside
matching curly brackets {} after while
keyword is the part of while
statement which is also called as
while
block or while
loop body.
Control flow diagram of while loop
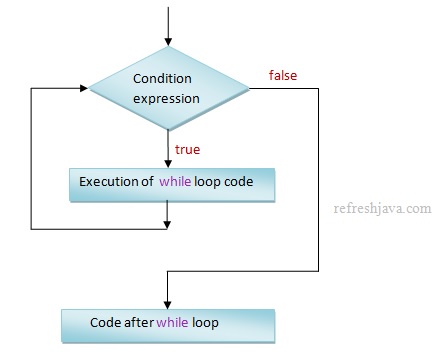
while loop program in Java
class
WhileLoop {public static void
main(String [] args) {int
num = 1;while
(num<=10) { System.out.println(num); num++; } System.out.println("After the end of while loop"
); } }
Output:
1
2
3
4
5
6
7
8
9
10
After the end of while loop
Can I test multiple conditions in while statement ?
Yes, you can test multiple conditions using && or || operator. For eg. while
(a>0 && b<10) is a valid statement.
Infinite while loop
If the condition in while
loop always returns as true
, it becomes an infinite loop.
while
(true
) {// code to be executed inside while loop
}
do while loop in Java
do while
is also a looping statement in java which allows to repeat the execution of one or more line of code as far as a condition is true
.
It's very similar to while
loop, the only difference is that
in do while
loop the statements inside do while
block executed first then condition expression is tested.
Therefore the code within the do
block is always executed at least once.
The syntax of do while
loop is :
do
{// code to be executed inside do while loop
}while
(condition);
Note the semicolon(;) at the end of while
statement, it's the part of declaration, programmer usually misses this while using the
do while
loop in java, which results in syntax error.
Control flow diagram of do while loop
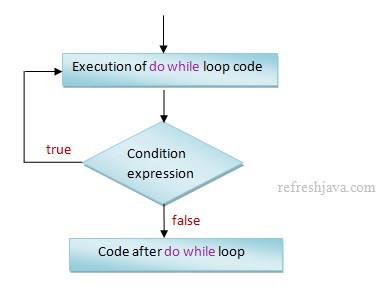
do while loop program in Java
class
doWhileLoop {public static void
main(String [] args) {int
num = 1;do
{ System.out.println(num); num++; }while
(num<=5); System.out.println("After the end of do while loop"
); } }
Output:
1
2
3
4
5
After the end of do while loop
Difference between while and do while loop in Java
In while
loop, the condition expression is checked first. If it is true
then the code inside the while loop get's executed otherwise it
won't be executed. While incase of do while
loop, the code inside loop is executed first then the condition is checked. If the condition is true
then the code inside the loop will be executed again otherwise it won't be executed. So in do while
loop the code inside the loop is executed at least once no matter
whether the condition is true
or false
which is not the case with while
loop.
When to use for, while and do while loop ?
When you know the code inside the loop should be executed for a fixed number of time, use for
loop. When you are not sure about repetition number,
go for while
loop, in this case if you want that your code
should be executed at least once, go for do while
loop. Just for an example if you want to print a table of a number, you know the iteration should be done for 10 times,
use for
loop and let us suppose you want
to know how many time a random integer number is divisible by to 2, go for while
loop eg. 256 is divisible 8 times, 12 is divisible 2 times only etc.
- Ensure all letter of while and do while keyword is small. While, WHILE, Do while, do While etc. are incorrect.
- Do not add semicolon(;) after while loop in case of while loop like while(condition); which will end the while loop there itself, but in case fo do while loop you must have to use semicolon.
- A common mistake that programmers sometimes do, they forget to change the while loop expression value inside the body of loop, which results as infinite while loop.
- Everything that can be done by for loop, can also be done using while loop and vice-versa. Select the right one as per the requirement.