Package import is a feature in java which allows us to reuse the classes available in a package. Java provides import
keyword to import
classes of another package inside your class. Once a class is imported, you can use the imported class anywhere in your class.
Package import basically makes availability of classes of that package in your class, so that you can use the functionalities of that classes. To access a class of another package, first you need to import that class or package in your class.
Importing packages allows us to import not only classes but also interfaces, enums etc as well. We are referring classes only in this tutorial, just for simplicity. This tutorial assumes that you already know how to compile and execute package program, if not, refer the package tutorial. The syntax of importing a package or class is :
// To import all classes of a package
import
packagename.*;// To import specific class of a package
import
packagename.classname;// To import all classes of a sub package
import
packagename.subpackagename.*;// To import specific class of a sub package
import
packagename.subpackagename.classname; Exampleimport
mypack.*;import
mypack.MyFirstPackageProgram;import
java.util.*;
The * wildcard is used to import all classes of a package. For example suppose if there are classes like PackageDemo1, PackageDemo2, PackageDemo3
etc inside a package
mypack
, then importing package mypack
using
* wildcard will import all these classes in your class. The * wildcard does not import classes of a sub package, they must be imported explicitly
as given above to import their classes.
The package import
statement must come after package name declaration and before the start of your class. If your class doesn't have a package declaration, then
import statement will be the first statement in your class.
Note: Accessibility of a class outside the package is also controlled by access modifier. You can only access it if it has an access modifier that allows it to be accessed outside. We will see access modifier in later tutorial.
Accessing classes of a package
To access the class of a package, you just have to import that class or package in your program. Let's understand this by the programs given below. In first program we are creating a class
MyFirstPackageProgram
in mypack package. The second program which is in another package accesses the MyFirstPackageProgram
class.
package
mypack;public class
MyFirstPackageProgram {public void
printMessage() { System.out.println("Inside printMessage method"
); } }
The program below which is in testpack
pacakge, shows how to import and use the above class. This program creates an object of above class and calls it's
printMessage
method.
package
testpack;import
mypack.MyFirstPackageProgram;// To import all classes of mypack package
// import mypack.*;
class
PackageImportProgram {public static void
main(String args []) { System.out.println("package import demo"
); MyFirstPackageProgram mfp =new
MyFirstPackageProgram(); mfp.printMessage(); } }
Now let's compile and execute the program. We need to compile MyFirstPackageProgram.java
first, then only we can compile and execute
PackageImportProgram.java
.
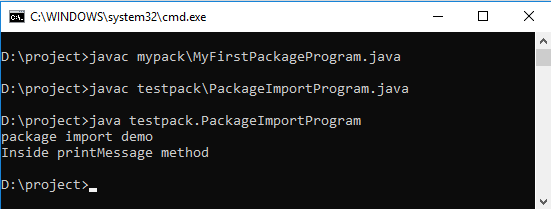
Output:
package import demo
Inside printMessage method
What if my program doesn't find the class in imported package ?
The program won't compile. It will throw compilation error.
Should I prefer to import particular class over importing all classes using * wildcard ?
Yes, It's good programming style to import only required classes over importing all classes using * wildcard.
Accessing class without importing the package
We can access the class of a package without importing it. In that case we will have to use the name of class with it's package name. For example the above program can be written without importing the class like below :
package
mypack;class
PackageImportProgram {public static void
main(String args []) { System.out.println("package import demo"
); testpack.MyFirstPackageProgram mfp =new
testpack.MyFirstPackageProgram(); mfp.printMessage(); } }
Output:
package import demo
Inside printMessage method
Accessing classes of built in java packages
To access the classes of built in java packages, you just have to import that class or package inside your program, once imported you can use that class anywhere in your program.
The program below shows how to access the class Arrays
of built in java packages java.util
.
import
java.util.Arrays;// To imports all classes of java.util package.
// import java.util.*;
class
SortArray {public static void
main(String args[]) {int
[] arr = {30,40,10,20,50}; Arrays.sort(arr);for
(int
i : arr) System.out.print(i +" "
); } }
Output:
10 20 30 40 50
Here Arrays
class is available in java.util package which has method sort
to sort an array.
Can I import multiple classes or packages in my class ?
Yes you can, in real world applications most of the classes needs to import multiple classes or packages.
Resolving class name conflict
If two package have class with same name, and you have imported both the packages in your program, then you might get the error while using the same name class in your class.
Let's understand this by an example. The package java.util
and java.sql
both has a class called Date
and
suppose you have imported both the packages in your program. Now if you use Date
class directly in your class, it will result in compilation error.
Now you can follow any of the approaches given below to avoid this error :
- If you want to access the
Date
class of a particular package in your program, then you can specifically import theDate
class of that package. For example to useDate
class ofjava.util
package, import like below :import
java.util.Date;import
java.sql.*; Date date =new
Date();// java.util.Date will be used
- If you want to access the
Date
class of both the packages, then you need to refer them using their packages name in your program like below :import
java.util.*;import
java.sql.*; java.util.Date date =new
java.util.Date(); java.sql.Date today =new
java.sql.Date(1000);
Static import in Java
Static import is a feature in java which allows us to access static
variables and methods of a class directly in our program without using their class name.
We just have to static import that member(variable or method), then we can use that member directly in our class. This feature is available from java version 5 and above.
We can import a specific static member or all the static members using static import. The code below shows how to import a specific and all members of Arrays
class of java.util
package.
// Imports only sort method of Arrays class
import static
java.util.Arrays.sort;// Imports all static member of Arrays class
import static
java.util.Arrays.*;
Static import program in Java
import static
java.util.Arrays.sort;class
SortArray {public static void
main(String args[]) {int
[] arr = {30,40,10,20,50}; sort(arr);for
(int
i : arr) System.out.print(i +" "
); } }
Output:
10 20 30 40 50
As you can see the sort
method is called directly without using the class name, since it's a public static
method in Arrays
class.
Note : You can not import a package using static import, it must be the class or static variable/method that can be imported using static import. For example using below import will result in compilation error.
import static
java.util.*;// compilation error