As in our computer we have directories to organize the files, similarly in java we have packages, used to organize the classes and interfaces files in java applications. Packages are very similar as directories which help us to write better and manageable code.
A package, as the name itself suggest is a group(pack) of similar types of classes and interfaces. Classes with similar type of functionalities are put together inside a package. It's the programmer who creates the package and adds the classes and interfaces inside that package.
A package can contain other types as well like enums, annotation types, subpackages. For simplicity we are referring classes and interfaces only, specially the classes in this tutorial.
The image below shows a hierarchy of different java elements where each element is a subset of next element. For example a method is a group of statements, similarly an application is a group of packages.

A package declaration looks like below :
package
packagename; Example:package
mypack;package
com.refreshjava.mypack;
Here package
is a keyword while packagename
is the name of package given by programmer. As per java the naming
of packages should be in small letters only.
Types of packages
Packages can be categorized in two types :
- User defined packages
- Built-in packages
This tutorial covers only user defined packages. For built-in packages refer built in packages tutorial.
User defined packages in Java
Packages defined by programmer is known as user defined packages. While working for an application/project you may need to create one or more packages in your application. Each packages may have one or more classes and interfaces inside it. Let's see how to create a user defined package.
Creating packages in Java
As mentioned above, a package is also a directory. Each package that you define is a directory in your computer. For example a package name mypack
will be a
directory mypack
inside your computer. Similarly a package name com.refreshjava.mypack
will have a directory structure as
com/refreshjava/mypack
, where directory mypack
will be inside refreshjava
directory which will be inside com
directory.
So to create a package, first you need to create a directory anywhere in your computer. You can also declare the package in your program first and then create the directory
with same name(as package name) in your computer. Let's create a directory mypack
inside the computer. In my computer it is created
inside D:\project
directory, you can choose your own directory. Currently mypack
is just an empty directory.
Once you have created the directory, you can use that directory as package name in your program. Remember the package name declaration must be the first statement in your program.
package
packagename;class
className { ... } Example:package
mypack;class
Test { ... }
Now let's create the class MyFirstPackageProgram.java
as given below inside the D:\project\mypack\
directory.
Java package program
package
mypack;class
MyFirstPackageProgram {public static void
main(String args []) { System.out.println("My first package program"
); printMessage(); }public static void
printMessage() { System.out.println("Inside printMessage method"
); } }
Notice here the package name and directory name is same. A package program must be saved inside it's own(package name) directory. Now let's see how to compile and execute the program.
Compilation and execution of package program
If you are not using tools like Eclipse, Netbeans etc, the compilation and execution of programs having package declaration is a bit tricky. To compile above program, open command prompt and
move to the directory D:\project
or the one that you have used. Execute the below command which will create the .class file
inside mypack
directory.
javac mypack\MyFirstPackageProgram.java
Now let's see how to run the above program. To run a package program you need to give the class name with it's package name while executing the program like below :
java mypack.MyFirstPackageProgram
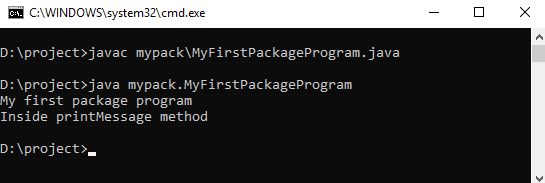
While compiling or executing the program, ensure that you are in D:\project
directory in command prompt or the one you have used for your package directory.
Output:
My first package program Inside printMessage method
It's mandatory to give the class name with it's package name while running the program since java looks for the .class file in same directory(package name).
Can a class have multiple package declaration ?
No a class can have only one package declaration. Declaring multiple package will result in compilation error.
Can multiple classes have same package declaration ?
Yes multiple classes can have same package declaration. All these class files must go inside same directory.
Creating .class files in separate directory
In above program we created the .class
file in mypack
directory but in real world applications, generally we use to create the .class
files in separate directory like classes
or build
directory.
It's good programming style to separate the source code and compiled code. To create .class
files in separate directory java provides -d
option
which is used with javac
command.
The -d
option with javac
command is used to specify the directory where the .class
files needs to be stored.
Create a folder classes
inside D:\project
directory in your computer and then execute the below command, it will create the .class
file inside classes
directory.
javac -d classes mypack\MyFirstPackageProgram.java
Here after -d
option you need to specify the directory name where to save the .class
file. Here it's classes
directory.
The .class
file will be generated inside D:\project\classes
directory, so ensure that it already exist.
After successful compilation java compiler will create a directory mypack inside classes directory.
If you want to save the .class
files in current or same directory, you can use .(dot) after -d
option which tells the
java compiler to save the .class
file in current directory.
Now to run the program you need to specify -classpath
or -cp
command line option with
java
command. This option tells the java virtual machine where to look for .class files.
java -cp classes mypack.MyFirstPackageProgram
// or
java -classpath classes mypack.MyFirstPackageProgram
Here after -cp
or -classpath
option you need to specify the directory name where to look for the .class
file.
In this case it's classes
directory where jvm will look for .class file. To get more detail about classpath refer Path and Classpath
tutorial.
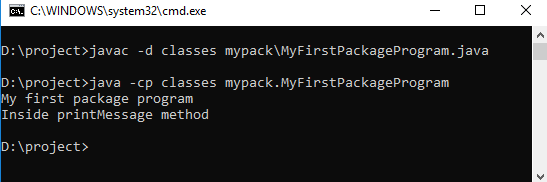
Output:
My first package program Inside printMessage method
What if JVM doesn't find the .class file at location given by cp/classpath option ?
JVM will throw error like "Could not find or load main class ... ".
Java package program with multilevel directory
The program below demonstrates the package having multilevel directory structure. Create the directory structure com/refreshjava/mypack
inside D:\project
directory and
then save below program inside that directory.
package
com.refreshjava.mypack;class
PackageDemo {public static void
main(String args []) { System.out.println("Package with multilevel directory"
); } }
Execute below commands to compile and run the program.
javac -d classes com\refreshjava\mypack\PackageDemo.java
java -cp classes com.refreshjava.mypack.PackageDemo
Output:
Package with multilevel directory
Advantages of Packages
The advantages of using packages are :
- Packages makes easy to search or locate class or interface files.
- Packages helps to avoid naming conflict. Classes with same name can exist in different packages.
- It also helps in controlling the access of one class inside other class.
- Packages helps in encapsulation of classes and interfaces.
- Packages helps to reuse the classes and interfaces.
- Java's predefined packages are available in rt.jar file in bin folder of JRE.
- Though you can use capital letters as well for package name but prefer to use small letters only.
- In organizations, generally packages are named in reverse order of domain names like
com.orgname
ororg.orgname
, for example com.refreshjava.mypack. - Programmer should avoid to use the word
java
for naming their packages likejava, java.xyz, java.refreshjava
etc, since java uses this word for java packages. - Every class is part of some package. If no package is specified, the class goes inside a default package(the current working directory) which has no name.