Java is an object oriented programming language. Classes and objects are the key concepts to understand object oriented programming. Java programming is based on these two terms. Let's understand what a class means in this tutorial and we will discuss about objects in next tutorial.
Programmatically a class is a group of variables, constructors, methods, blocks etc. These are optional components of a class. A class is defined using class
keyword
followed by class name. The name of the class is used to refer that class within or outside the class. The basic syntax of declaring a class is :
class
ClassName {// variables, constructors, // method declarations
}
Here class
is a keyword, used to define a class in java. ClassName
is the name of class, given by the programmer.
After the class name, it's the class body given inside { }. Variables, methods, constructors etc defined inside the balanced { }
after the class name are the part of that class.
Do we have any convention to write the class name ?
The convention is that the class name and any subsequent word in class name should start with upper case, for example
MyFirstProgram, HelloWorldProgram, Person, Bicycle
etc are valid class names of this convention.
Is class keyword case-sensitive ?
All keywords in java are case-sensitive and they must be in small letter. So you can not use class
keyword as Class, CLASS, clAss etc, it must be in small letters.
In object oriented world, think of a class as a blueprint or a design that basically describes how an object of a class will behave and what data(properties) it will contain inside it. The methods of a class defines the behavior of object while instance variables are the data of the object that it will contain inside.
Real time example of class
Some of the real world examples of class are Person, Vehicle, House, Tree, Dog
etc. For an example a Person
class have properties like name, age, height
etc.
and behaviors like walk, talk, eat, sleep
etc.
Now if you create an object of this class, it will have these properties and behaviors inside it. Once you created a class, you can create multiple objects of that class.
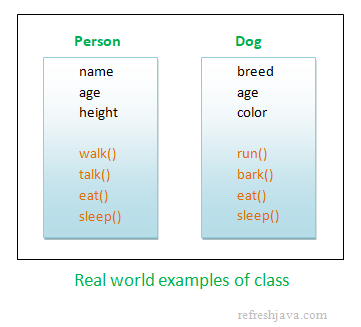
Can I give any name to my class ?
Yes, you can give any name to your class by following the identifier naming convention rules but giving a meaningful name is a good programming style.
For example class names like Abc
, XYZ
are valid names but not meaningful names while class names like MyFirstProgram, HelloWorldProgram, Person
are meaningful names.
Java Class Program
class
Person {// Instance variables, describes state/properties of object of this class.
String name;int
age;int
height;// methods, describes behaviors of object of this class.
public void
walk() { System.out.println("Hi my name is : "
+name+", age : "
+age+" year," +" height : "
+height+" cm. I can Walk"
); }public void
talk() { System.out.println("Hi my name is : "
+name+", age : "
+age+" year," +" height : "
+height+" cm. I can Talk"
); } }class
ClassDemo {public static void
main(String [] args) { Person p1 =new
Person();// Creating object of class Person
p1.name ="Rahul"
; p1.age = 20; p1.height = 170; p1.walk(); p1.talk(); } }
Save above program as ClassDemo.java
compile as javac ClassDemo.java
run as java ClassDemo
Output:
Hi my name is : Rahul, age : 20 year, height : 170 cm. I can Walk
Hi my name is : Rahul, age : 20 year, height : 170 cm. I can Talk
In above example we have created a class called Person
which is basically a prototype, describing that object of this class will have properties
as name, age, height
and will have behaviors as walk
and talk
. You can add more
properties(instance variables) and behaviors(methods) in your program. This example creates only a single object
of Person
class. You can create multiple objects of Person
class and assign different values inside it.
Should I create all classes in single file ?
No, that's not a good programming style. In real world programming mostly we use to create each classes in separate files in our application.
So next time when you are creating a class, think that you are creating a prototype which will tell that what properties(state)
and behaviors
will exist
inside the object of that class. This way you will get better idea to design your class.
A class is also called as a blueprint
or a template
or a design
or a Type
. These are just different words use to describe a class in java.
Who loads java classes in memory while execution ?
As soon as you run a program, the class-loaders available in java virtual machine loads classes in memory for execution. In java, these class-loaders are also a program.
Is it mandatory to define variables(instance or static variable) on top of a class ?
No, you can define such variables after the method or in end of a class but that's not a good programming style. You should always prefer to declare it at top since it makes a class more readable.
Can a class exist without main method ?
Yes, in real world programming only the starting class of your application(a group of classes) will have main method, all other classes won't have main method.
Should I focus more on real world aspect of class ?
As a programmer you should focus more on it's programming aspect rather than focusing on real world aspect, programming aspects like creation of class, creation of variables and methods inside the class, creation of objects of class etc.
The syntax of declaring a class given in this tutorial is the minimal one which is required. Some of the more keywords that can be declared along with class
keyword are
public, abstract, final, extends,
implements
. A class can also have another class, static blocks, enum
etc inside the body of it. We will discuss all these things in later tutorials.
- Every program must have at least one class, it can have more than one class as well.
- If there are multiple classes in a single program file, the file must be saved by class name declared with public keyword, if any.
- Every class(user defined or defined by java) in java is a non primitive data type.
- There should be only one main method having argument type as
String []
in a class. - If there are multiple non public classes in a single program file, conventionally the program file should be saved by class name having main method.