Encapsulation is another fundamental principal of object oriented programming. Many programmers specially beginners find it little difficult to understand what exactly the encapsulation is, so I will try to make it as simple as possible. The tutorial covers different details of encapsulation like what encapsulation is, example of encapsulation, advantages of encapsulation, abstraction vs encapsulation etc.
What is Encapsulation
Encapsulation is a process or technique that binds the data(member variables) and behavior(methods) together in a single unit. We call these unit's as class, interface, enum etc. The encapsulation process ensures that the data and behavior of a unit can not be accessed directly from other unit.
The first thing that comes in mind when we hear encapsulation is capsule
. The encapsulation is very similar as a capsule, as capsule binds it's medicine within it,
similarly in java the encapsulation process binds the data and behavior of a unit with that unit. You can think of encapsulation as a cover or layer which packages/binds related data and behavior together
in a single unit.
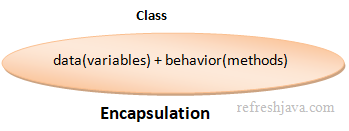
Real world example of encapsulation
As I already mentioned, one of the real world example of encapsulation is Capsule, as capsule binds all it's medicinal materials within it, similarly in java encapsulation units(class, interface, enums etc) encloses all it's data and behavior within it.
Another real world example can be your school or office bag. The bag contains different stuffs like pen, pencil, notebook etc within it, in order to get any stuff you need to open that bag, similarly in java an encapsulation unit contains it's data and behavior within it and in order to access them you need an object of that unit.
Encapsulation example program in Java
Every class, interface, enum etc that we create is an example of encapsulation, so let's create a class as given below to understand the encapsulation by an example :
class
Person { String name;int
age;void
walk() { }void
talk() { } }
Here the Person
class is an example of encapsulation. The variables(name, age
) and methods(walk, talk
) of the class is packaged/binded in a single unit
which is Person
class. Here, the encapsulation binds the implementation details of the Person class with it and hides it from other classes. If other classes want's to access these detail,
they need to create the object of Person
class in order to access it's data and behavior like below :
Person p =new
Person(); p.name ="Rahul"
; p.walk();
Similarly if you create an interface
or enum
, that is also an example of encapsulation. Now let's see another form or extension of encapsulation which is
data hiding.
What is Data Hiding
Data hiding or information hiding is a software development technique in object oriented programming which is used to hide the data from outside world. The purpose of data hiding is to protect the data from misuse by outside world. Data hiding is also known as data encapsulation.
Data Hiding = Hiding the Data
In java we use private
access modifier to protect/hide the data and behavior from outside world. For example if I declare the Person
class variables like below :
class
Person {private
String name;private int
age; }
Here we are hiding the variables from outside the class. These variables will be visible within the class only. This is basically what data hiding is wherein we hide the data of a class from outside world, so that other classes can not change/access these data directly.
class
ABC { Person p =new
Person(); p.name ="Rahul"
;// compilation error, as name attribute won't be accessible here
}
Now the question is what if other classes wants to read and write these data ?, because if other classes can't use this class data, then this class won't be that useful.
So in order to allow these data to be accessed from outside, we can create getters(getXXX())
and setters(setXXX())
methods for these variables like below.
Data hiding program in Java
class
Person {private
String name;private
int age;public
String getName() {return
name; }public String
getAge() {return
age; }public void
setName(String name) {this
.name = name; }public void
setAge(int
age) {this
.age = age; } }
The getters
and setters
methods are used to read and write the variable value of a class respectively. In data hiding we generally allow other
classes to access/modify the variables through getters
and setters
only in order to avoid misuse of data.
Furthermore you can apply more control in setters method so that no one can set any unwanted value to avoid misuse. For example if you don't want to allow other classes to set any blank or
null
value in name
field you can add below logic :
public void
setName(String name) {if
(name ==null
|| name.equals(""
)) {throw new
IllegalArgumentException("Name can not be null or empty"
); }this
.name = name; }
Similarly you can apply logics in other setter
methods as well if needed, in order to protect any illegal data. Applying such logics to avoid any illegal data basically increases
the security of your application.
Why do we use data hiding
The main reason of using data hiding is security. As we use private
modifiers with our variables, we can store critical informations in such variables which will be
visible within the class only, no one else can access them directly. As we also apply conditions in setter methods whenever needed, it also increases the security so that no one can set any
illegal data for any misuse.
How to achieve encapsulation in Java
Though you are already achieving encapsulation by creating classes, interfaces, objects etc but if you want to create a fully encapsulated class in java, follow below things :
- Declare all your member variables with
private
access modifier. - Create
public
getters
andsetters
methods of those variables in order to allow access of those variables from outside the class.
Advantages of Encapsulation
- Security: Encapsulation helps to secure your code, since it ensures that other units(classes, interfaces etc) can not access the data directly.
- Flexibility: Encapsulation makes your code more flexible, which in turn allows the programmer to change or update the code easily.
- Control : Encapsulation gives the control over data stored in the member variables. For example, you can control what value can be set in age of a
Person
given in above program without breaking the code. - Reusability: Encapsulated code or unit can be reused anywhere inside the application or across multiple applications. For example, if you have
Person
or any other class in your application you can reuse that class wherever needed. - Maintainability: Encapsulated units are easy to maintain as you can locate or change the things easily.
Difference between abstraction and encapsulation in Java
Though both are quite interrelated and plays role in one another, but to list down some of the differences :
- Abstraction focuses more or less on hiding the internal detail/logic while encapsulation focuses more on protecting/hiding the data from misuse.
- Abstraction is more or less design/modeling level concept while encapsulation is more or less implementation level concept.
- Encapsulation focuses more on access modifiers to hide/protect the information while abstraction focuses more on abstract methods to hide the implementation details.
- Using encapsulation, you can deny access to the fields of a unit either by declaring their getters method as private or by not defining the getters method at all.
- Java beans are good example of encapsulation in java, as they contains private variables with public getters and setters methods.
- As soon as you start increasing visibility of data and behavior with access modifier, you start loosing their bindings with encapsulation units.
- Using encapsulation you can make your class read only or write only. To make read only, declare only getters method of your variables and for write only, declare only setters method of your variables.