Sometime you may require to select one block of code from multiple block of code. To address such requirements java provides switch
statement which
allows java programmers to select one block from multiple
block of statements. The syntax of switch
statement is:
switch
(expression) {case
constant-1:// code of case 1 block
break
;case
constant-2:// code of case 2 block
break
;case
constant-3:// code of case 3 block
break
; . . . . . .case
constant-N:// code of case N block
break
;default
:// code of default block
}
switch
statement evaluates it's expression and then selects the matching
block. The working of switch
statement is very similar to if-else if
ladder, which means the same
thing can be achieved using if-else if
statement.
Does switch statement moved directly to matching case ?
No, It starts matching from top to bottom, the one that is matched, the code of that block is executed.
A programmer should remember below points while using switch
statement in java.
- The expression used in a
switch
statement should be of typebyte
,short
,char
,int
,enums
andString
(Java SE 7 and above). Wrapper classesCharacter
,Byte
,Short
andInteger
can also be used. - A programmer can have any number of
case
statements within aswitch
block. Eachcase
statement must be followed by thevalue
to be compared to and a colon(:). - Each case
value
must be unique. Duplicating a casevalue
will result in compile time error. - The type of
value
for everycase
must be same as data type ofswitch
statement expression. Each casevalue
must be a constant or a literal. You can not use variables, for examplecase
num1
,case
num2
are incorrect declaration. - There can be multiple lines of code(statements) for each
case
. - When a
case
is matched, all the statements of thatcase
will execute until abreak
statement is reached. - When a
break
statement is reached, theswitch
block terminates, and the flow of control jumps to the next line after theswitch
block. - Use of
break
statement is optional, If not used, all the cases(including codes inside it) after the matchingcase
will execute until abreak
statement or end ofswitch
statement is reached. - The default case in
switch
statement is optional. It can be used to perform any tasks when no cases is matched.
Control flow diagram of switch statement
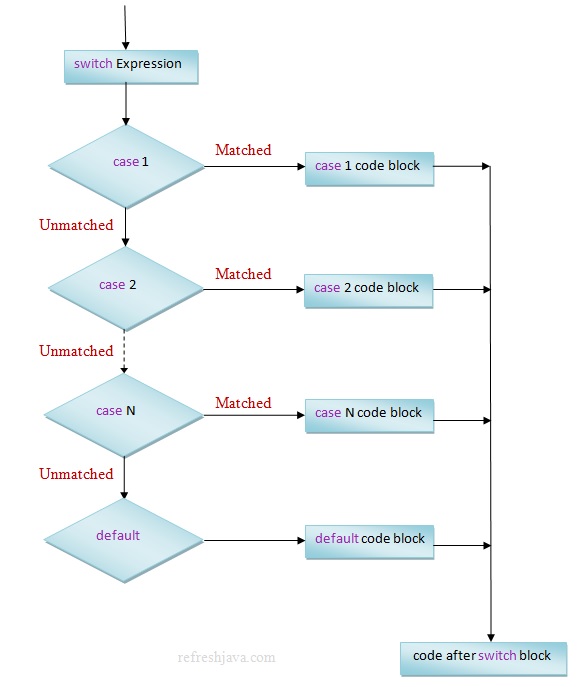
The above diagram shows the control flow of switch
statement assuming that you have used break
statement in every
case
of switch
block
switch case program in Java
class
SwitchStatement {public static void
main(String [] args) {int
dayNo = 4; String dayName;switch
(dayNo) {case
1: dayName ="Sunday"
;break
;case
2: dayName ="Monday"
;break
;case
3: dayName ="Tuesday"
;break
;case
4: dayName ="Wednesday"
;break
;case
5: dayName ="Thursday"
;break
;case
6: dayName ="Friday"
;break
;case
7: dayName ="Saturday"
;break
;default
: dayName ="Invalid day"
;break
; } System.out.println("day name = "
+dayName); } }
Output:
day name = Wednesday
Programmer can decide whether to use if
-then-else if
or a switch
statement on the basis of readability
and the expression that the statement is testing. One example is, if
-then-else if
should be preferred when you want to test ranges of value
like a>0 && a<10
while switch
statement should be preferred to test single value like for a==5
execute this,
for a==4
to execute that etc.
- Conventionally you should use default statement in last, though it's not mandatory but prefer to follow this.
- Keywords are case-sensitive, can not use like Switch, SWITCH, switcH etc. Every letter should be small letter.
- return statement can also be used inside switch cases, once executed the flow will be exited from the method itself.
- Expressions like a+b, a+b*c etc. can also be used in switch statement.