String is one of the most widely used data type by java
programmers. Things that we normally use in conversations like "hello"
, "refresh java"
,
"Hello world"
, "It's easy to learn java"
, name of a person, address of a person etc are some of the examples
of string in java.
In java a string is basically a sequence of characters, for
example the string "hello"
is a sequence of characters
'h', 'e', 'l', 'l', 'o'
. Similarly each string in java is a sequence of characters. Java language provides
String
class to create and manipulate strings in your programs. This class is defined in java.lang
package which
comes automatically in your java software(JDK or JRE).
Since a class in java acts as a non primitive data type, so String
is also a non
primitive data type. You can use String
class as a data type to store string values. The Syntax of declaring a string is :
String var_name ="refresh java"
; String var_name =new
String("Hello World"
);
Here String
is the data type, var_name
is the name of variable which is given as per the programmers choice and the value of
string variable is given inside ""
. You need to use the double quotes(""
) to create string values in java.
Which approach I should prefer to declare a string, ""
or new
keyword ?
Though you can use any of the above approach but creating a string using new
operator is not commonly used and is not recommended as it creates an extra object
inside heap memory.
String class program in Java
class
StringDemo {public static void
main(String args[]) {// Creating String variables
String strVar ="refresh java";
String strVar2 =new
String("Hello World"
); char[] charArray = {'h', 'e', 'l', 'l', 'o'
}; String strVar3 =new
String(charArray); String strVar4 = strVar;// Printing the values of String variables
System.out.println("strVar = "
+strVar); System.out.println("strVar2 = "
+strVar2); System.out.println("strVar3 = "
+strVar3); System.out.println("strVar4 = "
+strVar4); } }
Output:
strVar = refresh java
strVar2 = Hello World
strVar3 = hello
strVar4 = refresh java
Java string memory allocation
String values in java are stored inside a special memory known as string pool or string constant pool which exist inside the heap memory. This memory is used to
allocate space for string values only. String values given inside ""
in your program are created inside string pool. To understand memory allocation of strings, let's consider
we have following string variables declared inside a program.
1. String s1 ="refresh java"
; 2. String s2 =new
String("Hello World"
); 3. String s3 =new
String("hello"
); 4. String s4 ="refresh java"
; 5. String s5 ="hello"
;
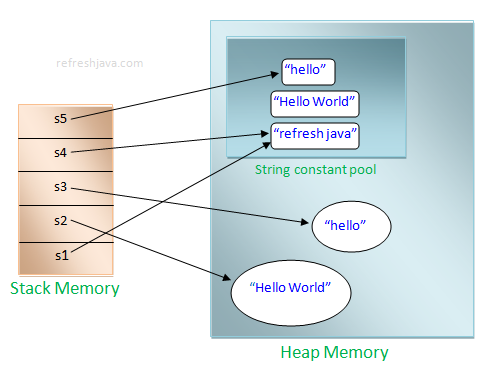
Let's understand how java assigns memory for above strings variables.
- Line 1, java checks if string literal
"refresh java"
is already in string pool or not, since it's not there, java creates this string literal in string pool and assigns the reference(address) of this ins1
. - Line 2 is creating object
s2
usingnew
keyword, so java will create it inside the heap memory with value as"Hello World"
and it will also create the string literal("Hello World"
) inside the string pool as well. - Line 3 is also creating object
s3
usingnew
keyword, so it will also be created inside the heap memory with value as"hello"
and the same string literal("hello"
) would also be created inside string pool. - Line 4, java again checks the string literal
"refresh java"
inside the string pool, since it's already there in pool, so it won't create it again. Java assigns the reference of existing string literal ins4
. - Line 5, java again checks the string literal
"hello"
inside string pool, since it's already there in pool, so it won't create it again. Java assigns the reference of existing string literal ins5
.
Why java uses a special memory for string ?
Since String is one of the most useful data type, to improve the memory usage and application performance java usage string pool memory as it doesn't create unnecessary or duplicate objects, instead it uses the existing string objects.
What is string literal in java ?
A series of characters in your program that is enclosed in double quotes(""
) is a string literal. For example "hello world"
,
"refresh java"
, "java is easy to learn"
are string literals in java. Whenever it encounters a string literal in your code, the compiler
creates a String object with its value in string constant pool. String literals are string constants in java.
How can I check if two string variables or literal have same reference(address) ?
You can use ==
operator to compare if two string variables or literals have same address. For example s1==s2
, s1==s4
etc.
You can refer next tutorial for more details about this.
String concatenation in java
This is also used very often by java programmers. String concatenation is a way of concating(adding) two or more string values. Java provides the feature of adding two or more string variable or
values using plus(+
) operator. For example you can add two string values "refresh"
and "java"
as
"refresh"
+"Java"
which results as a new string "refreshJava"
. Java also provides the
concat
method in String
class to concat two string variables or values. You can refer next tutorial about this method.
Java program to concatenate two string
class
StringConcatDemo {public static void
main(String args[]) { String s1 ="refresh"
; String s2 ="Java"
; s1 = s1+s2; s2 ="refresh"
+ s2 +" tutorial"
; System.out.println("s1 = "
+s1); System.out.println("s2 = "
+s2); } }
Output:
s1 = refreshJava
s2 = refreshJava tutorial
String is immutable in Java.
Immutable simply means something that is not changeable, once a string value in java is created, you can not change that value. For example in above program the code s1+s2
will create a new string "refreshJava"
and assigns the reference of this in s1
but the original string
"refresh"
will still be there in string pool memory, that is why string in java is immutable.
Why string is immutable in Java ?
Since immutable strings can not be changed, the same string can be referenced in multiple programs or applications which significantly improves the memory usage and application performance.
- String pool, string constant pool and string literal pool and string intern pool are same things.
- Digits can also be stored as string in java, for example String str = "12345"; is a valid string.
- The '+' operator, which performs addition on primitives (such as int and double), is overloaded to perform concatenation on String objects. The compiler, internally uses the append method of StringBuffer/StringBuilder class to implement string concatenation.