Java has already defined some packages and included that in java software, these packages are known as built-in packages or predefined packages. These packages contains a large number of classes and interfaces useful for java programmers for different requirements. Programmers can import these packages in their program and can use the classes and interfaces of these packages in that program.
Built-in packages comes automatically in your jdk/jre download. These packages comes in the form of jar files. By unzipping the jar file you can see the
packages available in that jar. For example if you unzip rt.jar
file available in lib
folder of JRE, you can see the directory java
which contains packages like lang, io, util, sql
etc.
There are many built-in packages available in java. In this tutorial we will see some of the built-in packages and how to use their classes in our program. Some of the inbuilt packages in java are :
- java.awt : Contains classes for creating user interfaces and for painting graphics and images. Classes like
Button, Color, Event, Font, Graphics, Image
etc are part of this package. - java.io : Provides classes for system input/output operations. Classes like
BufferedReader, BufferedWriter, File, InputStream, OutputStream, PrintStream, Serializable
etc are part of this package. - java.lang : Contains classes and interfaces that are fundamental to the design of Java programming language. Classes like
String, StringBuffer, System, Math, Integer
etc are part of this package. - java.net : Provides classes for implementing networking applications. Classes like
Authenticator, HttpCookie, Socket, URL, URLConnection, URLEncoder, URLDecoder
etc are part of this package. - java.sql : Provides the classes for accessing and processing data stored in a database. Classes like
Connection, DriverManager, PreparedStatement, ResultSet, Statement
etc are part of this package. - java.util : Contains the collections framework, some internationalization support classes, properties, random number generation classes. Classes like
ArrayList, LinkedList, HashMap, Calendar, Date, TimeZone
etc are part of this package.
As mentioned above, you can unzip the jar files to see the list of all java packages or you can refer this link to see the list of all packages available in java 11.
The package java.lang
is automatically imported to every program that we write, that is why we don't need any import
statement in our programs
for using classes like String, StringBuffer, System
etc. Except java.lang
, other packages must be imported first in your program to use the classes and
interfaces available in that packages. To get complete detail about how to import packages in java, refer package import tutorial.
Java awt package(java.awt)
Java AWT(Abstract Window Toolkit) package contains classes and interfaces used to develop graphical user interface or window based applications in java. Java AWT components
are platform-dependent since they uses operating system resources to create components like textbox, button, checkbox
etc. The program below creates a frame containing a label
using java.awt
classes.
Java awt package program
import
java.awt.Frame;import
java.awt.Label;class
JavaAWTExample {// Declaring constructor
public
JavaAWTExample() { Frame fm =new
Frame();//Creating a frame
Label lb =new
Label(" Welcome to refresh java"
);//Creating a label
fm.add(lb);//adding label to the frame
fm.setSize(300, 200);//setting frame size
fm.setVisible(true
);//setting frame visibility as true
}public static void
main(String args []) { JavaAWTExample awt =new
JavaAWTExample(); } }
Here Frame
and Label
are classes defined in java.awt package. Frame
class is used
to create frame while Label
class is used to create label. AWT package is rarely used today because of it's platform dependency and heavy-weight nature.
Swing
is the preferred API over AWT for developing graphical user interface in java.
Output:
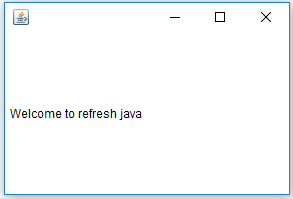
Java lang package(java.lang)
This packages contains the fundamental or core classes of java language without which it won't be possible to write program in java. This package is automatically imported to each program, which means we can use the classes of this package directly in our program.
The program below shows how to use the Math
class of java.lang
package which provides many different methods for different
mathematical operations.
Java program of demonstrating use of java.lang package
class
JavaLangExample {public static void
main(String args []) {int
a = 20, b =30;int
sum = Math.addExact(a,b);int
max = Math.max(a,b);double
pi = Math.PI; System.out.printf("Sum = "
+sum+", Max = "
+max+", PI = "
+pi); } }
Output:
Sum = 50, Max = 30, PI = 3.141592653589793
As you can see we are able to use Math
and System
classes defined in java.lang
package without any
import statement, it's because java.lang
package is imported implicitly.
Java io package(java.io)
Java IO(Input/Output) package provides classes and interfaces for handling system(computer, laptop etc) input and output operations. Using these classes programmer can take the input from user and do operations on that and then display the output to user. Generally input is given using keyboard/keypad. We can also do file handling(read/write) using the classes of this package.
The program below uses the Console
class of java.io
package to take the input from user and then print that input to user's screen(command prompt or
any other terminal used).
Java program of demonstrating use of java.io package
import
java.io.Console;class
JavaIOExample {public static void
main(String args []) { Console cs = System.console(); System.out.println("Enter your name : "
); String name = cs.readLine(); System.out.println("Welcome : "
+name); } }
Once you run this program, it will ask for your name and then it will display that name in console window.
Output:
Enter your name :
Rahul
Welcome : Rahul
Java util package(java.util)
Java util package provides the basic utility classes to java programmers. It is one of the most useful package for java programmers, it helps them to achieve different types of requirements easily by using it's predefined classes.
The program below uses Arrays
class of this package to sort an array of integers. The Arrays
class provides many api's(methods) for
different array requirements.
Java program of demonstrating use of java.util package
import
java.util.Arrays;class
JavaUtilExample {public static void
main(String args []) {int
[] intArray = {10,30,20,50,40}; Arrays.sort(intArray); System.out.printf("Sorted array : %s",
Arrays.toString(intArray)); } }
Output:
Sorted array : [10, 20, 30, 40, 50]