Generally each line of code in a program executed only once. Sometime we come to a situation where in we want to repeat the execution of one or more line of code more than once.
To address such requirements java provides looping statements. The looping statements are special symbols
which asks the computer to repeat the execution of instructions written inside these statements as far as a given condition is true
.
Java provides below looping statements to repeat
the execution of statements in a program.
-
for
loop -
while
loop -
do while
loop
For an example let us imagine, we have to print digits from 1
to 10
, one approach could be, we can write
a program which includes 10
print statements, but what about if you need to print
digits from 1
to 100
or more ?. Java looping statements can help you to accomplish the same in
an easy way. Java for
loop
can be used to repeat execution of statements for a fixed number of times or till
a condition is true
. The basic syntax of for
loop is :
for
(initialization; condition; increment/decrement) {// code to be executed inside for loop
}
Everything given inside () after for
loop are optional expressions which performs specific tasks. Initialization expression is used to initialize variables,
objects etc. for example int
i = 0
, int
count=10
etc.
Condition expression is used to test the condition, for example i<=10
, count>0
etc.
Increment/decrement expression is used to change in variable or object value, for example i++
, count--
etc.
Everything that comes inside matching curly brackets {} after for
keyword is the part of for
statement which is also called as
for
block or for
loop body.
How for loop works
- Initialization expression is the first expression which get's executed as the loop begins and it is executed only once.
- Then condition expression is evaluated, it's a boolean expression which returns either
true
orfalse
. - If the condition expression returns as
true
,- Statements inside the body of
for
loop is executed. - Then the increment/decrement expression is executed.
- Again, the condition expression is evaluated.
If it returns as
true
, again the statements inside the body offor
loop is executed and then increment/decrement expression is executed. This process goes on until the condition expression returns asfalse
.
- Statements inside the body of
- If the condition expression returns as
false
,for
loop terminates.
Control flow diagram of for loop
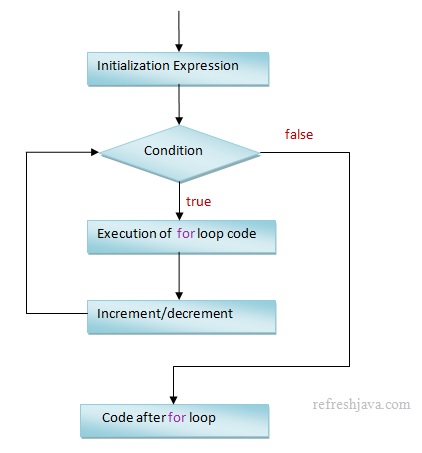
for loop program in Java
class
ForLoop {public static void
main(String [] args) {for
(int
num=1; num<=10; num++) { System.out.println(num); } System.out.println("After the end of for loop"
); } }
Output:
1
2
3
4
5
6
7
8
9
10
After the end of for loop
Can I use multiple variables in for loop expressions ?
Yes you can use multiple variables in for
loop expressions. For eg. for
(int
x=0, y=10; x<10 && y>0; x++, y-- )
is a valid java for
loop. You can initialize multiple variables of same data type only in initialization expression.
Infinite for loop
What if the condition expression written in for
loop statement always returns as true
, the body of for
loop will keep executing, this is basically called as infinite for
loop.
class
InfiniteForLoop {public static void
main(String [] args) {for
(int
num=1; num>0; num++) { System.out.println(num); } } }
In above program, num>0
will always return true
since num
is increasing in every iteration. So for
loop
will keep executing infinite number of times. If you are running this program inside command prompt, just press ctrl + c
to exit from execution.
In for
loop syntax, all the expressions are optional, programmer can leave all of them as blank. If all
of them leaved as blank, this again becomes infinite for
loop.
for
( ; ; ) {// code to be executed inside for loop
}
- Ensure that all letters in for keyword is small, can not use For, FOR foR etc.
- Do not forget to add semicolon(;) between different expressions of for loop, missing that will result in compile time error.
- Do not add semicolon(;) after for loop like for(...); which will end the for loop there itself.
- Variables declared in initialization expression are accessible within for loop body. You can not access outside of for loop body.
- Ensure condition expression is correct, sometime programmers make mistake in this, which results as infinite for loop.