Runtime and compile time, these are two programming terms that are more frequently used in java programming language. The programmers specially beginners find it little difficult to understand what exactly they are. So let's understand what these terms means in java with example.
In java running a program happens in two steps, compilation and then execution. The image below shows where does compile time and runtime takes place in execution of a program.
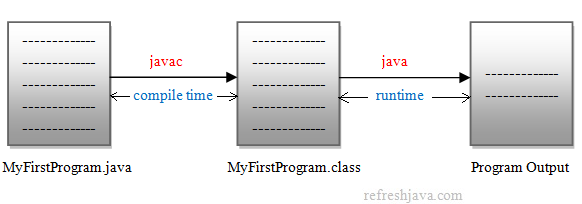
What is compile time in Java
After writing the program, programmer needs to compile that
program. As soon as the programmer starts compiling the program
using
javac
compiler, the compile time gets started, and it ends when either
a .class file is generated after successful compilation or an
error is thrown in compilation. In other way we can say, the
process of compiling a program is referred as compile time.
For an example if you wrote a program and saved it as
MyFirstProgram.java
, Once you start compiling this program using
javac
command as
javac MyFirstProgram.java
,
the compile time gets started and it ends when a .class file as
MyFirstProgram.class
is generated or any error is thrown in compilation.
What happens at compile time in java ?
At compile time, the java compiler(javac) takes the source code(.java file) and checks if there is any syntax, type-checking or any semantic errors inside the program. If there is no error, the compiler generates a .class(bytecode) file for that .java file. If there is any compilation error, java compiler displays that error in command window(eg cmd).
What is compile time error in java ?
If a program element(class, method, variable, statements etc) is not written as per it's syntax in java, the compiler throws error for that element while compiling the program. We call these errors as compile time errors as these errors are detected at compile time by the java compiler.
Does java compiler generates .class file even if it throws compilation error ?
No, it will not generate .class file, it will only display the compilation error in console(eg. cmd) window.
Let us see compile time error by an example. The most common mistake that beginners do is, they forget to add semicolon(;) at the end of a statement which results as a compilation error while compiling the program.
class
MyFirstProgram {public static void
main(String [] args) { System.out.println("first statement"
)// missing semicolon(;)
System.out.println("second statement"
); } }
Once you compile above program as javac MyFirstProgram.java
, it will display a compilation error in console window like below :
Compile time Error:
MyFirstProgram.java:3: error: ';' expected System.out.println("first statement") // missing semicolon(;) ^ 1 error
What is Runtime in Java
As soon as the programmer starts executing the program using
java
command, runtime gets started and it ends when execution of
program ended either successfully or unsuccessfully. In other way the process of running a program is known as
runtime.
For an example if you wrote a program and saved it as
MyFirstProgram.java
. After compilation when you execute the command
java MyFirstProgram
for running the program, runtime gets started and it ends when either
the output of program is generated or any runtime error is thrown.
What is runtime error in java ?
Errors which comes during the execution(runtime) of a program are known as runtime errors. If a program contains runtime error, it won't run successfully, rather that error will be displayed in command window(eg. cmd) at the time of execution.
Let us suppose if you wrote a program MyFirstProgram.java
like below, After compilation when you run the below
program using java MyFirstProgram
command, it
will throw a runtime error.
class
MyFirstProgram {public static void
main(String [] args) {int
num1 = 10;int
num2 = 0; System.out.println(num1/num2);// Runtime error: Divide by zero exception
} }
Runtinme Error:
Exception in thread "main" java.lang.ArithmeticException: / by zero at MyFirstProgram.main(MyFirstProgram.java:5)
Difference between runtime and compile time
Compile time is a process in which java compiler compiles the java program and generates a .class
file. In other way, in compile time
java source code(.java file) is converted in to .class file using java compiler. While in runtime, the java virtual machine loads the .class
file in memory
and executes that class to generate the output of program.
Difference between compile time error and runtime error
Compile time errors are the error that comes while compiling the program whereas runtime errors are errors that comes at the time of execution(run-time) of the program. An example of
compile time error is "not adding a semicolon(;) at the end of a statement"
in your java program while "dividing a number by zero"
is an example of runtime error.
- Without compilation you can not run the program, compile first then run.
- The output of a program is generated in runtime, not in compile time.
- If you made any changes in program after compilation, you need to compile the program again to see the changes in output.