This tutorial will cover brief description about some of the basic or common terms used in a java programs. The detail description of each terms will be given in it's respective tutorials. For beginners it's necessary to understand these terms in order to start writing program in java.
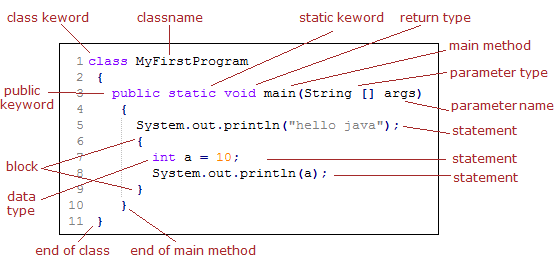
Keywords - Every programming language defines a set of words which has a predefined meaning in that programming language. These words are known as keywords. You can not use these words for variable names, method names, class names or any other identifier names in your program, since these are reserved words. Java has also defined some words which has a predefined meaning in java programming language, java programmers can not use these words for naming their identifiers in a program. These reserved words are known as keywords in java.
For example, in statement
, int
a = 10int
is keyword which represents the
data type of variable a
. You can not use
int
keyword as your variable, method or class names. Similarly the words
class, public, static, void
are java keywords in above program. To see the list of all java keywords refer
the table given in Identifier Naming Convention section .
// Following are incorrect declaration since final and switch are keyword in java.
int
final = 20;// final can not be used as variable name
class
switch {}// switch can not be used as class name
Are java keywords case sensitive ?
Yes, keywords in java are case sensitive. All letters of keyword must be small.
What if I use keywords as my variable, class or method name ?
Your program won't compile, java compiler will throw compilation error.
class - The class
is a keyword in java
which is used to define a class. In java every program must have a class. A class contains
set of methods and variables. After class
keyword
programmers need to write the name of the class which is used to refer that class within or outside the class. In above program
MyFirstProgram
is the name of class, everything that is inside balanced {} after
the class name are the part of class. Refer classes in java tutorial to get a complete detail about class in java.
Statement - A statement is similar to a sentence in english language. As sentences makes a complete idea, similarly java statement makes a complete unit of execution. In above program line 5,7,8 are statements.
Method - A method is set of
statements that performs specific task or call other methods. A method
has a name and a return type. The name of the method is used to refer that method within or outside the class. A class can have multiple methods. In above
program main
is the method name. Everything that comes in balanced { }
after method name are the part of method. Every java program must
have a main
method if it needs to be run independently. The main
method is the starting point of
execution of a program in java. Refer methods in java tutorial to get more detail about methods.
block - A block is a group of zero or more statements. it starts with curly braces { and ends with balanced }. All statements inside balanced { } is the part of block. A block is generally used to group several statements as a single unit. A block can have another block inside it. Blocks does not have a name, they are just logical grouping of statements inside { }. Refer static and instance initializer blocks in java to get more detail about blocks in java.
public - The keyword public
is an access modifier
that decides the visibility or accessibility of a member. Variables or
methods declared with public
keyword can be accessed outside the class. Since main
method is called by
JVM at the time of program execution that is why it
must be declared as public
, otherwise JVM won't be able to find the main method in your program and your program will not execute.
Refer access modifiers in java tutorial to get more detail about access modifiers in java.
static - The static
is a keyword
in java. A method or variable declared with static
keyword can be called without
creating the object of that class. Since
JVM calls the main
method without creating the object of class, that is why it must be declared as
static
, otherwise JVM won't be able to call the main
method. Refer
static keyword tutorial to get more detail about static
keyword in java.
return - The return
is also a keyword
in java. It is used to return value from the method to the caller of
the method. Every method must have a return type, if it's not
returning any value then the return type of that method must be void
. Since main
method doesn't return any value,
that's why it's return type is void
. Refer return statement tutorial to get more
detail about return
keyword in java.
Variable and data type - Variable in java is
very similar to variable in mathematics, used to store value. Data type of a
variable defines what type of data that variable can store. In above program
a
is a variable and int
is it's data type, which means
a
can contain only integer type of value. Refer variable in java tutorial to get more detail about variables.
Parameter - A parameter is
special kind of variable which receives the value
from the caller of the method. A parameter can be used within the method in which it is declared. In above example args
is a
parameter of type String
array. Any argument passed to program while running the program
is stored in args
parameter. Refer this tutorial to get more detail about parameters in java.
System.out.println() - It is used
to print the output(any string or variable) of a program on console. Anything passed to println
method
will be printed on console. A console is basically a window or
terminal where you can pass input to program or print the output
of a program. Command prompt(cmd) in windows is an example of console.
After reading this tutorial you may have got some understanding about basic terms in java program but still you may have number of questions, don't worry you will get to know more detail about these terms in later tutorials.
- All letters of
main
method that needs be called by java JVM must be small, can not use Main, mAin, maiN etc. - Every method name must be preceded by a return type.
Use
void
in case method is not returning any value. - Every method name must be followed by (). A method may or may not have parameters.
- The name of parameter
args
in main method can be as per programmers choice, you can use other name as well. The only thing is that it's type must be of String array.