Once the execution of a loop statement in a program starts, it keeps repeating the execution of loop instructions as far as a given condition is true
.
Sometimes it may be required to break the execution flow in between. To break the execution flow of a loop, java provides a keyword called as
break
. It can be used
inside for
, while
, do while
and switch
statements only. As soon as
a break
statement encountered in a loop, the execution of that loop get's terminated and the control
flow jumps to the next statements after the loop body. It is mostly used along with conditional statements(if
,
else
). The syntax of break
statement is:
break;
Control flow diagram of break statement
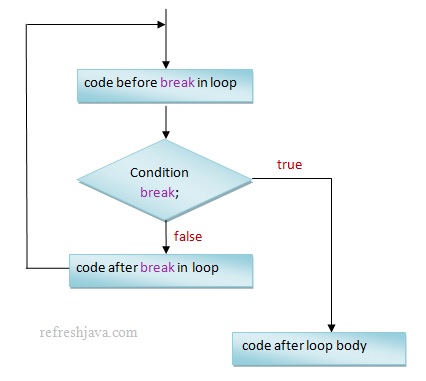
Once the break
statement is executed, the remaining statements which are after the break
and within the loop are skipped.
Java break
statement has two forms, labeled and unlabeled. Following program shows unlabeled
break
statement.
break Statement program in Java
class
BreakStatement {public static void
main(String [] args) {for
(int
i=1; i<=5; i++) {if
(i==4) {break
; } System.out.println("i = "
+i); } System.out.println("line after for loop"
); } }
Output:
i = 1
i = 2
i = 3
line after for loop
In above example, as soon as the value of i
becomes 4
, the break
statement is executed and the
control flow jumps to next line(System.out.println("line after for loop");
) after the for
loop, that is why value
4
and 5
is not printed.
What if I use break statement outside of loop or switch statement ?
Your program won't compile, it will throw compilation error.
Does break Break Out all loops ?
No, It breaks only the current loop in which it is used.
If break
statement is used inside any inner loop, it breaks only inner loop once it is executed and the execution flow moves to the next line after inner loop. The
outer loop will be executed as usual.
class
InnerLoopBreakStatement {public static void
main(String [] args) {for
(int
i=1; i<=3; i++) { System.out.println("Outer loop i = "
+i);for
(int
j=1; j<=5; j++) {if
(j==3) {break
; } System.out.println("j = "
+j); } } System.out.println("line after outer for loop"
); } }
Output:
Outer loop i = 1
j = 1
j = 2
Outer loop i = 2
j = 1
j = 2
Outer loop i = 3
j = 1
j = 2
line after outer for loop
Labeled break Statement
A label is an identifier, from java SE 5 and above we can declare a set of statements with a label
identifier. Labeled break
statement allows programmer to break the execution of label statements.
class
LabeledBreakStatement {public static void
main(String [] args) { firstLable:for
(int
i=1;i<=3;i++) { System.out.println("Outer loop i = "
+i); secondLabel:for
(int
j=1;j<=5;j++) {if
(i==2 && j==2) {break
firstLable; } System.out.println(" j = "
+j); } } System.out.println("line after labeled break statement"
); } }
Output:
Outer loop i = 1
j = 1
j = 2
j = 3
j = 4
j = 5
Outer loop i = 2
j = 1
line after labeled break statement
In above example as soon as the value of i
and j
becomes 2 the statement break firstLable
is executed which breaks the firstLable
statements and the execution moves to the next line which is
System.out.println("line after labeled break statement");
See java switch statement tutorial for how break
statement is used with switch
statement in java.
- break statement cannot be used outside of a loop or a switch statement. If used, it will throw a compile time error.
- Ensure every letter of break keyword is small, can not use Break, BREAK, breaK etc.
- You can also place curly braces {} after label identifier to declare statements inside it.