Bytecode is one of the most common java programming terms which is used more often while learning java programming. As a beginner sometime it's quite confusing to understand what exactly the bytecode is. In this tutorial let's understand what bytecode is in simple terms and also the differences with some other type of programming terms to make it more clear.
What is bytecode in Java
In java when you compile a program, the java compiler(javac
) converts/rewrite your program in another form/language which we call as bytecode.
The .class file that is generated after compilation is nothing else, it's basically the bytecode instructions of your program.
The word bytecode and .class
are used interchangeably, so if someone says bytecode, it simply means the .class file of program.
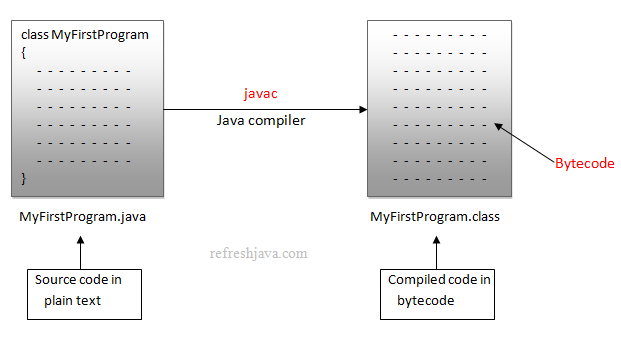
At runtime Java virtual machine takes bytecode(.class
) as an input and convert this into machine(windows, linux, macos etc)
specific code for further execution.
So bytecode in java is just an intermediate representation/code of your java program in the form of .class
file.
We call it intermediate representation/code because it lies between source and machine code.
Source code → Bytecode → Machine code
Bytecode is also one of the java's magic because
this along with java virtual machine(JVM) makes java platform independent and a secure language. It becomes platform independent because
you can run the bytecode generated on one machine on any other machine without the need of original .java
file,
all you need is the jvm/jre software installed on that machine. For example .class
file generated on windows machine can be run on linux machine.
Bytecode also makes java secure because it can be run by java virtual machine only.
Also any explicit edit in .class
file breaks down the class which means
you will not be able to run your program through the edited .class
file.
Does computer understands bytecode ?
No, Computer doesn't understand bytecode directly. At runtime the bytecode is converted into machine specific code by java interpreter. This machine code is then executed by the computer processor to generate the output of program.
Is bytecode human readable ?
No, bytecode is not human readable.
Who generates bytecode in java ?
It's the java compiler(javac).
Is bytecode machine dependent or independent code ?
Bytecode is machine independent code, it can be run on any machine, you just need the JRE/JVM of corresponding machine.
How does java bytecode works
The image below shows the execution flow of a program in java.

In java a program(.java file) is compiled first using java compiler(javac
). After successful compilation the bytecode(.class file) of that program
is generated. Now when you run the program using java
command, the java virtual machine(JVM) loads the .class file inside memory and
then converts(by java interpreter) the instructions of .class file into machine executable code and finally executes that machine code in order to generate the output of program.
Does same bytecode is generated of a program if compiled on different operating system ?
Yes, Same bytecode is generated of a program even if compiled on different operating system.
What is write once run anywhere(WORA) in Java
Write Once Run Anywhere(WORA)
is a feature in java which says write your program once and then execute it anywhere(OS). In java it is
achieved with the help of bytecode. Programmer needs to write the
program once and can execute the .class
file generated from it anywhere and any number of times.
What is the use of bytecode in Java
The main reason to use bytecode in java is to make java platform independent and secure. The bytecode of a program can be run on any machine which is why java is called platform independent. Also the use of bytecode makes java a secure language, any external edit in bytecode of a program fails it's execution.
It's the bytecode of a program which is used for running a java program. At runtime java virtual machine loads the bytecode of a program inside the memory and converts into machine code and then executes that machine code.
Advantages of Bytecode
Some of the advantages of bytecode in java are :
- Bytecode helps java to be a platform independence language. This is one of the main advantage of bytecode in java.
- It helps java to be a secure programming language, as it can be run by java virtual machine only. Also at runtime bytecode is validated by bytecode verifier, any explicit edit in bytecode fails it's execution.
- It makes java portable which helps to achieve "
Write once, run anywhere
" feature. It simply means you need to write the program once, and execute it anywhere(OS), all you need to have is the .class of that program generated on any machine. - Generally the size of bytecode is less than the source code, therefore it is easy and fast to transport them over the network/internet.
- Another advantage of bytecode is, one can exchange it to others without disclosing the logic implemented in the program.
Bytecode vs Machine code
Bytecode is an intermediate code, it can not be run directly on any machine(computer, laptop etc). It must be converted into machine code before it can be executed. In java, java interpreter(part of jvm) converts the bytecode into machine code.
Machine code is native or low level code that machine can understand and execute. They are generally binary or hexadecimal instructions which can be executed directly by computers CPU.
Difference between source code and bytecode
Soucre codes are basically the code written inside a program by following the syntax of a programming language. In java,
the code written inside a .java
file are known as source
codes. While bytecode is the code which is generated after compiling the java program. In java the .class
files are referred as bytecodes.
What is bytecode verifier in java
Bytecode verifier is a special java runtime module/program which verifies the bytecode(.class
) before it get's executed. Bytecode verifier traverses the
bytecodes and checks if the bytecode
loaded are valid and do not breach java's security restrictions. Think of bytecode verifier as a gatekeeper which ensures that code passed to the java interpreter is in a correct state to be
executed and can run without the fear of breaking the Java interpreter.
As soon as you run a program, the classloader(part of jvm) loads the .class file in memory. After that the bytecode verifier verifies if the bytecode is valid or not. If it's valid then only it is passed to java interpreter for further execution.
- The .class files are usually smaller in size than source code, that means it also gets compressed.
- As bytecode doesn't allow any external editing, it's also secure to transport them over the network.
- Bytecode is a machine code for java virtual machine but not for actual machines(OS).
- We can use
javap
disassembler likejavap MyclassName.class
to see some information stored in .class file. - Programmer should not focus much on understanding information stored in .class file.
- Bytecode makes java different from other languages like C, C++ where source code is directly converted to machine code