Sometime you may require that for a particular repetition of a loop, you don't want to execute few or all instructions of loop body. To solve such requirements
java provides continue
keyword which allows programmers to skip the
current iteration of a loop and move to the next iteration, which
means if you want to skip the execution of code inside a loop
for a particular iteration, you can use continue
statement. Only the code written after the continue
statement
will be skipped, code before the continue
statement will execute
as usual for that iteration.
continue
statement can be used only inside for
, while
and
do while
loops of java. In case of break
statement the control
flow exits from the loop, but in case of continue
statement, the
control flow will not be exited, only the current iteration of
loop will be skipped. It is mostly used along with conditional statements(if
,
else
). The syntax of continue
statement is:
continue;
Control flow diagram of continue statement
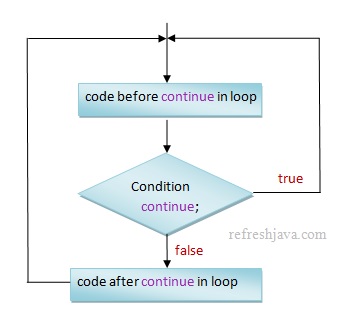
Once continue
statement is executed inside for
loop, the control flow moves back to for
loop to
evaluate increment/decrement expression and then condition expression.
If continue
statement is executed inside
while
and do while
loop, the control flow jumps to condition expression for evaluation.
Java Program of continue Statement
class
ContinueStatement {public static void
main(String [] args) {for
(int
i=1; i<=5; i++) {if
(i==4) {continue
; } System.out.println("i = "
+i); } System.out.println("line after for loop"
); } }
Output:
i = 1
i = 2
i = 3
i = 5
line after for loop
As you can see in above program, for i==4
the continue
statement is executed which skips the current iteration and move to the next
iteration, that is why value i = 4
is not printed.
If continue
statement is used inside any inner loop, it skips current iteration of inner loop only, the
outer loop will be executed as usual.
class
InnerLoppContinueStatement {public static void
main(String [] args) {for
(int
i=1; i<=3; i++) { System.out.println("Outer loop i = "
+i);for
(int
j=1; j<=2; j++) {if
(i==2) {continue
; } System.out.println(" j = "
+j); } } System.out.println("line after outer for loop"
); } }
Output:
Outer loop i = 1
j = 1
j = 2
Outer loop i = 2
Outer loop i = 3
j = 1
j = 2
line after outer for loop
Can I use break and continue statement together in a loop ?
Yes you can definitely use both statements in a loop.
Labeled continue Statement
A Labeled continue statement allows programmer to skip the current iteration of that label.
Java Program of labeled continue statement
class
LabeledContinueStatement {public static void
main(String [] args) { firstLable:for
(int
i=1;i<=3;i++) { System.out.println("Outer loop i = "
+i); secondLabel:for
(int
j=1;j<=3;j++) {if
(i==2) {continue
firstLable; } System.out.println(" j = "
+j); } } System.out.println("line after end of firstLable "
); } }
Output:
Outer loop i = 1
j = 1
j = 2
j = 3
Outer loop i = 2
Outer loop i = 3
j = 1
j = 2
j = 3
line after end of firstLable
In above program as soon as the value of i
becomes 2, the continue firstLable;
statement is executed which moves the execution flow
to outer loop for next iteration, that is why inner loop doesn't print anything for i=2
.
Java return Statement
As the return
keyword itself suggest that it is used to return something. Java return
statement is used to return some
value from a method. Once a return
statement is executed, the control flow
exited from current method and returns to the calling method. The syntax of return
statement is:
return
expression;return;
Who get's the value that is returned by method ?
The caller of the method. This value can be assigned in some variable.
The return
statement given above has two forms:
- First that returns a value. To return a value, just place the value or expression after
return
keyword. for eg.return
10
,return
a+b
,return
"refresh java"
etc. The data type of the returned value must match the return type of it's method declaration. - Second that doesn't returns a value. To use, just place
return
keyword. When the return type of method is declared asvoid
, you can use this form ofreturn
as it doesn't return a value, or you can also leave out to usereturn
keyword for such methods.
Java Program of return statement
class
ReturnStatement {public static void
main(String [] args) {int
sum = sum(10,20); System.out.println("Sum = "
+sum); }public static int
sum(int
a,int
b) {return
a+b; } }
Output:
Sum = 30
In above program, a
and b
are integers, so a+b
will also be an integer which is equivalent to return
type of method sum which is also an int
.
- continue statement cannot be used outside of a loop, if used it will result in compile time error.
- Use continue statement more precisely inside while and do while block, if not used precisely, it may result as infinite loop
- Ensure all letter of continue keyword is small, can not use Continue, contiNue, CONTINUE etc.
- Ensure all letter of return keyword is small, can not use Return, RETURN etc.
- return keyword can be used anywhere inside method, but not outside method.