Objects are key concepts of java programming, since java is an object oriented programming language. In object oriented programming, program focuses on objects rather than logics. This tutorial explains different details about an object in java.
In real world you can see many examples of object around yourself: a person, your dog, laptop, mobile, bicycle etc. Real world objects have two characteristics, state
and behavior
. For example a person have state like name, age, height
etc and behavior like walk, talk, eat
etc while a dog have state
like breed, age, color
etc and behavior like run, bark, eat
etc.
Similarly software object also consist of two characteristics: state
and behavior
. The state
of an object
is defined by the instance variables of a class while behavior is defined by the methods of class. So object in java is a collection of data(instance variables) and methods that acts
on those data. Programmatically an object is an instance of a class.
So next time when you are creating a class, think of two things about it's objects. "What possible states can this object have?" and "What possible behavior can this object perform?".
This way you will get better idea to design your class. You can think of a software object like below which holds it's state
and behavior
together with itself.
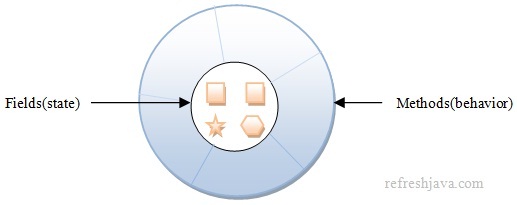
The Syntax given below shows the most basic way of creating an object of a class in java. There are other ways as well but we will not discuss that here.
ClassName objName = new
ClassName();
Here ClassName
is the name of class whose object need to be created and objName
is the name of object, given by the programmer. The object name
should be unique and should follow the convention given in identifier naming convention. The keyword new
is used to create object in java. Using the ()
with ClassName
calls the constructor of that class to
create and initialize the object in memory. We will discuss about constructors in later sections. Let's assume we have two classes Person
and MyFirstProgram
,
code given below shows how to create an object of these classes :
// Creates an object of Person class with name as obj.
Person obj =new
Person();// Creates an object of MyFirstProgram class with name as mfp.
MyFirstProgram mfp =new
MyFirstProgram();
How can I access properties and methods of an object ?
You just have to apply dot(.) operator with object name followed by property/method name to access the properties and methods of an object. You can also change the value of a property of an object using the same operator. Refer program given below to see the use of this operator.
What if I access object name only ?
In java object name is a reference, so accessing the name will return the reference(address) of that object in memory.
Object program in Java
class
Person {// Instance variables, describes state/properties of object of this class
String
name;int
age;int
height;// methods, describes behaviors of object of this class
public void
walk() { System.out.println("Hi my name is : "
+name+", age : "
+age+" year," +" height : "
+height+" cm. I can Walk"
); }public void
talk() { System.out.println("Hi my name is : "
+name+", age : "
+age+" year," +" height : "
+height+" cm. I can Talk"
); }public void
eat() { System.out.println("Hi my name is : "
+name+", age : "
+age+" year," +" height : "
+height+" cm. I can Eat"
); } }class
ObjectDemo {public static void
main(String [] args) {// Creating object p1 of Person class
Person p1 =new
Person(); p1.name ="Rahul"
; p1.age = 20; p1.height = 170; p1.walk(); p1.talk(); p1.eat();// Creating another object p2 of Person class
Person p2 =new
Person(); p2.name ="Rohit"
; p2.age = 30; p2.height = 180; p2.walk(); p2.talk(); p2.eat(); } }
Save above program as ObjectDemo.java
compile as javac ObjectDemo.java
run as java ObjectDemo
Output:
Hi my name is : Rahul, age : 20 year, height : 170 cm. I can Walk
Hi my name is : Rahul, age : 20 year, height : 170 cm. I can Talk
Hi my name is : Rahul, age : 20 year, height : 170 cm. I can Eat
Hi my name is : Rohit, age : 30 year, height : 180 cm. I can Walk
Hi my name is : Rohit, age : 30 year, height : 180 cm. I can Talk
Hi my name is : Rohit, age : 30 year, height : 180 cm. I can Eat
Here p1 and p2 are objects of Person
class. Once the code line Person p1 =
executed, java constructors
creates the object new
Person();p1
in heap
memory and assigns the default values of the instance variables for this object. After that code lines p1.name, p1.age and p1.height
modifies those default values
of the instance variables for this object only. Similarly p2 is also created and assigned the values of instance variables. All the objects of a class are created inside heap memory.
You can think of object p1
like below.
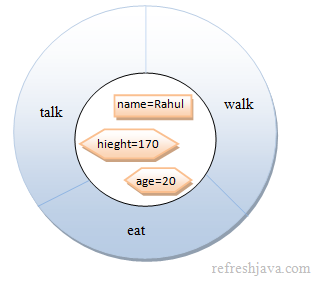
Who creates objects in java ?
It's the constructor that creates object of a class inside heap memory. We will see about constructors in later sections.
At runtime each object that you have created in your program will be allocated space in heap memory. Each object will have it's own copy of instance variables and methods defined inside the class. Changing the value of a instance variable for one object will not change the value of that variable for other object.
Can an object exist without a class ?
No, an object can never exist without a class. Every object must have a class which defines it's type.
What happens to objects when program execution completed ?
As soon as the execution of a program completes, all it's objects are destroyed/removed from memory.
Should I focus more on real world aspect of object ?
You should focus more on it's programming aspects rather than on real world aspects, programming aspects like creation of object, accessing it's fields and methods, accessing object of other class etc.
- Every object in java has a unique ID, that is not known to external programmers. JVM uses this id internally to identify each object uniquely.
- Word object and instance are used interchangeably.
- Fields, properties, attributes, state of an object refers to same thing which is generally instance variables of a class.
- An object can contain another object inside it.
- Only non-static variables and methods belong to an object of a class.