Sometime as a programmer you want to use a set of conditions where in you want that if your first condition is false
then only move to check the next condition,
and if that is also false
then only move to check the next condition and so on. To address such scenarios java provides if
then else if
statement. You can specify another condition with else if
statement which is evaluated only when it's prior
if
or else if
condition is false
.
If the else if
condition returns
true
,
the else if
block will be executed,
if it returns false
, computer will move to next else if
statement and
evaluates the condition expression. This process goes on until any of the expression returns
true
or all the
else if
statements are finished. Once any of the expression returns
true
, code inside that conditional statement is executed and
all other condition statements after that are not executed. If all conditional statements returns
false,
then final else
block(if any) will be executed.
The syntax of
if
-then-else if
statement is:
if
(condition) {// code to be executed inside if block
}else if
(condition-1) {// code to be executed inside this else if block
}else if
(condition-2) {// code to be executed inside this else if block
} . . .else if
(condition-N) {// code to be executed inside this else if block
}else
{// code to be executed inside else block, it's optional
}
The last else
statement in if
else-if
ladder is optional, it's programmer's choice whether to use or not.
What is if-else if ladder statement in Java ?
The if
.. else if
statement given above is also called as else if ladder which is mostly used when programmer wants to
select one block of code among many.
In if
.. else if
ladder only one block(the one whose condition is true
) of code is executed.
Can I use else if statement without if statement ?
No, you can not use else if
statement without if
statement in java. The
else if
must be used along with if
statement.
Flowchart of if...else if statement
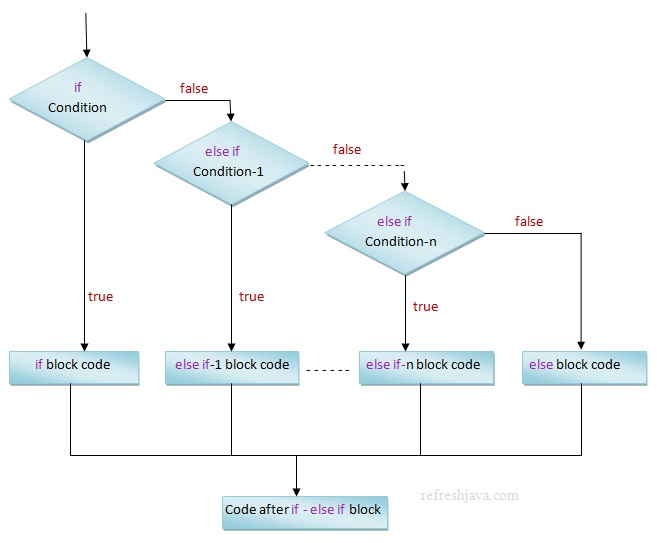
if .. else if program in Java
public class
IfElseIfStatement {public static void
main(String [] args) {int
num1 = 20, num2 = 20;if
(num1 > num2) { System.out.println("num1 is greater than num2"
); }else if
(num1 == num2) { System.out.println("num1 is equal to num2"
); }else
{ System.out.println("num1 is less than num2"
); } System.out.println("code to be executed after else if ladder"
); } }
Output:
num1 is equal to num2
code to be executed after else if ladder
Can we use if inside else if in Java ?
Yes, we can use if
statement inside else if
statement, the program will run successfully.
Nested if-else statement in Java
Java allows programmer to place if
else
block inside another if
or else
block. This is
called as nested if else
statement. Programmer can do any level of nesting in program which
means you can place if
else
block inside another if
or else
block up to any number of times.
Inner if
block is executed only when outer if
condition is true.
if
(condition-1) {// code to be executed inside first if block
if
(condition-2) {// code to be executed inside second if block
if
(condition-3) {// code to be executed inside third if block
. . . . . . . . . . }else
{// code to be executed inside else block
}// code to be executed inside second if block
}// code to be executed inside first if block
}else
{if
(condition-4) {// code to be executed inside this if block
} }
Nested if else program in Java
public class
NestedIfElseStatement {public static void
main(String [] args) {int
num1 = 20;if
(num1 >= 10) {if
(num1 < 100) { System.out.println("num1 is a double digit number"
); }else
{ System.out.println("num1 is not a double digit number"
); } }else
{ System.out.println("num1 is less than 10"
); } System.out.println("code after nested if else block"
); } }
Output:
num1 is a double digit number
code after nested if else block
Can we use if inside if in Java ?
Yes, this is known as nested if
in java. Refer the example above.
Can we use if inside else in Java ?
Yes, this is also known as nested if
else
in java. Refer the example above.
- Ensure there is space between else & if, it should not be elseif.
- The else if is also part of conditional or decision making statements.
- If no curly brackets {} is used with else if keyword, only first line of code after else if keyword will be considered as part of else if statement.