Object oriented programming(OOP) is a programming paradigm or style of writing program. As the term object oriented programming itself suggests, writing programs in this style is
oriented or based on the concept of objects. This programming style says that, view everything as an object, be it you, your car, dog, chair, house, remote etc where each objects have it's own
data
and behaviors
.
The data
of an object is basically the properties of that object while behavior
is something, what that object does. For example a person object have
properties like name, age, height
etc while behaviors like walk, talk, eat
etc.
So in OOP, computer programs are basically a representation of object's data and behavior. The data of an object in a program is given using variable(instance variable) while behavior is given in form of methods(instance methods). Each object can change it's data and behavior as the user wants and it can also interact with other object through it's behaviors. The object oriented programming has following concepts and features :
- Object
- Class
- Interface
- Object oriented programming features :
- Abstraction
- Encapsulation
- Inheritance
- Polymorphism
What is Object in Oops
An object can be think of a real world entity, for example person, dog, chair bicycle, television etc are objects. These objects have two things, state(attributes or properties or data) and behavior.
To give you an example, a person have state like name, age, height
etc and behaviors like walk, talk, eat
etc. Identifying the state and behavior of an object is a
great way to start thinking in terms of object-oriented programming.
Programmatically an object is a software bundle of state and behavior. State of an object is defined by instance variables and behavior of an object is defined by methods. Think it in another way, the instance variables of your program defines the state of object while the instance methods of your program defines the behavior of object.
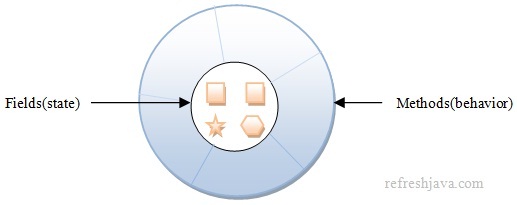
Object holds their state and behavior within them. To get more details about objects like how to create object in java programming, how to use them etc, refer objects in java tutorial.
What is Class in Oops
All object-oriented programming languages support the concept of class. A class is basically a predefined instructions which tells that what data and behavior the object of that class will have. The behavior of an object is given in the form of methods in a class while the data is given in the form of fields. We also call these predefined instructions, a template or design.
For example if we need to create a class for person objects, we can do something like below :
class
Person { String name;int
age;void
talk() { System.out.println("I can talk"
); }void
walk() { System.out.println("I can walk"
); } }
Here the Person
is a class which is basically describing that the object of this class will have fields/data like name
and age
while behaviors like
talk
and walk
. To get more detail about classes, refer class in java tutorial.
What is Interface in Oops
Interfaces are very similar as classes except that they only declare the set of behaviors of an object, they don't define(implement) those behaviors. The implementation or definition of those behaviors are given by the classes implementing that interface.
More programmatically, Interfaces declares only the signature of the methods and not their implementations. The methods that are declared in the interface are implemented by the implementing classes. The code below shows how we create an interface in java :
interface
Shape {public void
draw();public double
getArea(); }
Here Shape
is an interface which declares the behaviors draw
and getArea
. If a shape class like circle, rectangle etc implements this interface, they will
provide the implementation of these behaviors. Refer interface in java tutorial for more about interfaces.
What is Abstraction in Oops
Abstraction is a process of hiding the internal details or implementations from the user and showing only relevant or abstract(brief or short) information to user. Abstractions says that, show only those information of an objects behavior which is essential or useful for the user and hide all other information which is not essential for them.
A real world example of abstraction could be your TV remote. The remote has different functions like on/off, change channel, increase/decrease volume etc. We use these functionalities just pressing the button. The internal mechanism of these functionalities are abstracted(hidden) from us as those are not essential for us to know, this is what abstraction is. Abstraction says that, focus on what an object does rather than how it does.
In java abstraction is achieved using abstract classes and interfaces as these types contains set of abstract behaviors(method), the internal working of these behaviors are defined by some other classes. Refer abstraction in java tutorial for more detail about abstraction.
What is Encapsulation in Oops
The process of binding or enclosing(as in a capsule) the state(instance variables) and behavior(methods) together in a single unit is known as encapsulation. You can think of encapsulation as a cover or layer which binds the data and behavior together in a single unit. This layer prevents the access of data/behavior of a class outside the class.
To take a real world example, we can think of capsule, as capsule binds all it's medicinal materials within it, similarly in java encapsulation units(class, interface etc) encloses all it's data and behavior within it.
In java every class, interface or object that we create is a form of encapsulation. Each class contains it's information(variables and methods) associated with it, other classes needs to create object of this class in order to access these information. Refer encapsulation in java tutorial for more detail about encapsulation.
What is Inheritance in Oops
This is another fundamental principle of object oriented programming. Inheritance is a mechanism by which object of one class is allowed to inherit the features(fields and methods) of another class. This feature basically allows us to reuse the existing code.
The class that inherits the feature of another class is known as subclass or child class and the class whose feature is being inherited is known as super class or parent class. By inheriting the parent class the child class get's the access of fields and methods of parent class.
To take a real world example we can consider parent child relationship. The properties of parents like hands, legs, eyes etc and the behaviors like walk, talk, eat etc are inherited in child, so child can also use/access these properties and behavior whenever needed.
Java provides extends
keyword to inherit the feature of an existing class. The basic syntax of inheriting a class is :
class Aextends
B {// Here Class A will inherit the features of Class B
}
Here class A is subclass or child class while class B is superclass or parent class. Here Class A will inherit the features of class B which means class A will be able to access the variables and methods of class B. Refer inheritance in java tutorial for more detail about inheritance.
What is Polymorphism in Oops
This is another fundamental principle of object oriented programming. The word polymorphism is made from two words,
Poly
which means many
and morphism
which means forms or types
, so the word polymorphism means many forms
.
Polymorphims in java is a mechanism in which the object or it's behavior can have many different forms.
More programmatically in java a child class object can be assigned in any of it's parent class type which means the object of child class can take the form or act as any of it's parent class in inheritance hierarchy. We call such objects as polymorphic object.
To take a real world example, we can consider ourself. As a person we have many different forms like student, teacher, player, father/mother etc. The same person can be a teacher as well as a player, so we can say person object is polymorphic in nature. To understand the polymorphism in detail refer polymorphism in java tutorial.
- Java is an object oriented programming language but not purely object oriented.
- Some of other object oriented programming languages are C++, C#, Go, Lisp, Ruby, Smalltalk etc.
- Smalltalk language is purely object oriented language.
- Some of the non object oriented programming languages are ALGOL, BASIC, Pascal, C etc.
- If a language supports oops features, we call it object oriented programming language.