It's easy to start writing program in java. To write your first java program, open an editor like notepad, notepad++ or any other editor and write bellow line of code.
class
MyFirstProgram {public static void
main(String [] args) { System.out.println("refresh java"
); } }
The program given above is a very basic java program which will just print a string message refresh java
in console after execution. We will
understand different terms and their meaning used in above program in next tutorial. The image given below shows
the above program written in Notepad editor.
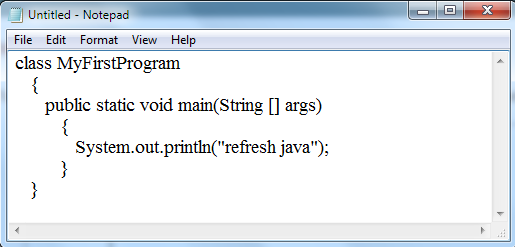
After writing the code in editor, the next step is to save the file as per the convention given by java which says the file name should be same as the class name
with .java
extension. So let's save the above program file as MyFirstProgram.java
.
In above program the name of class is MyFirstProgram
.
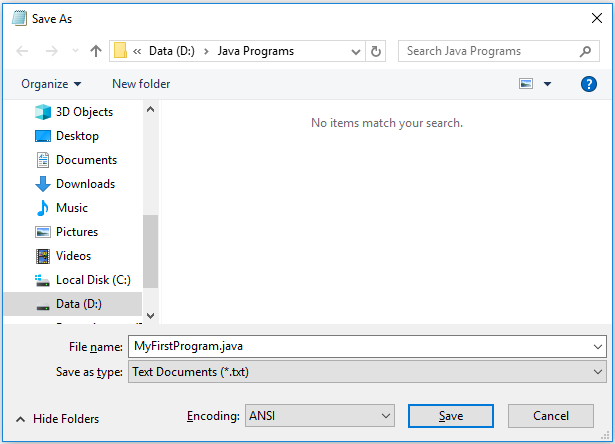
Is it mandatory to save file name as class name ?
No, if it doesn't contains a class declared along with public
keyword. If it contains a public class then it must be saved with
public
class name. It's good to follow the convention given by java. The keyword public
is an
access modifier.
What does a .java file contain ?
A .java file contains the source code written in java of a program.
How to compile java program
After saving the file, the next step is to compile the java program using javac
command. Before compiling ensure that Path
variable for java is set in your
system. To compile above program, open a terminal(eg. CMD in windows)
and move to the directory where above program
is saved. In my case program is saved inside D:\JavaPrograms
directory. Now execute the following command :
javac MyFirstProgram.java
// Don't forget to add .java extension while compiling.
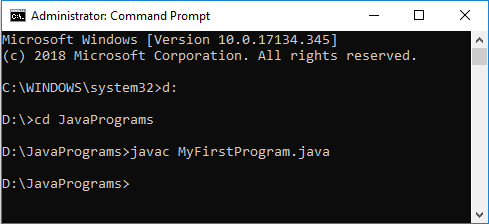
Once programmer executed above command, java compiler will check
for any syntax error in the program, if it doesn't find any
error, a .class
file as MyFirstProgram.class
will be generated at
the same location where program is saved. In successful compilation, compiler does not display any message on command prompt instead a .class
file is generated.
Remember you need to write program file name with .java
extension after
javac
command while compiling the program.
What is .class file ?
A .class file is a compiled code of your java program into another language which is called Bytecode. It's an intermediate representation of your java program. Refer Bytecode tutorial for more detail about bytecode in java.
How to run java program
Once the .class
file is generated, the next step is to run it using java
command which uses only the .class
file of that program at the time of execution. Execute following java
command in the same directory where .class
file is
available to run the above java program.
java MyFirstProgram
// Don't add .class extension while running.
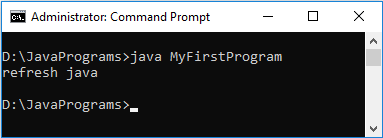
As you can see in the screenshot, once you executed the above command, it displayed the
output("refresh java
") in CMD. Remember you need to write .class
file name without .class
extension after java
command while running the program.
If you are in a directory other than your classpath(.class
) directory, you can use
-cp
or -classpath
attribute of java to provide the classpath directory while executing the program. The current directory in the image below is D:\Softwares
while the
.class
file is in D:\JavaPrograms
. Using the -cp
or -classpath
variable we can execute
the program MyFirstProgram
available in D:\JavaPrograms
directory. Refer Path and Classpath tutorial to know about
classpath in java.
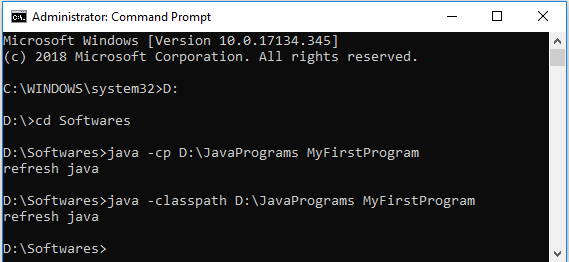
Where do Java programs begin execution ?
From main method, it's the starting point of program execution.
Does java uses .java file while running the program ?
No, it uses only .class file while running the program.
What if I delete the .java file after generation of .class
file, can I run my program ?
Yes you can, as .java
file is not needed at runtime. Only .class
file is needed while running the program.
The next tutorial will give you a brief introductions of different terms that we have used in above program and will cover some of the other aspects of a program as well.
- In java, generally every program must have at least one class, it can have more than one class.
- There should be only one main method having argument type as
String []
in a class. - If there are multiple classes in a program, It must be saved by class name having public access modifier if any.
- Every starting { must have a balanced } for it.